Universal Windows Platform (UWP) provides a common app platform on every device that runs Windows 10. The core APIs in UWP are the same on all Windows devices – including Desktop PC, Mobile Phone, XBox, Hololens, IOT devices and others.
You can target specific device capabilities of a device family with extension SDKs, but you don’t have to do that if you are only using core APIs. Those core APIs include a very impressive set of UI capabilities. What that means is that you can create a UWP application using C# and XAML which will run on an ARM based processor on Raspberry Pi – because Windows 10 IOT Core runs on that.
Universal Windows Platform (UWP) has come a long way. I decided to dust off my good old Raspberry Pi 2B and take it for a spin last weekend.
The goal was simply to create a Hello World UWP application, deploy it on Windows 10 IOT running on Raspberry Pi (RPi), without using any device specific extension SDKs. Here is the stack –
- Universal Windows Platform App (C#/XAML)
- Raspberry Pi Model 2B
- Windows 10 IOT Core
- Syncfusion Essential Studio 18.1.0.42
- Visual Studio 2019
Create a simple UWP application in Visual Studio 2019.
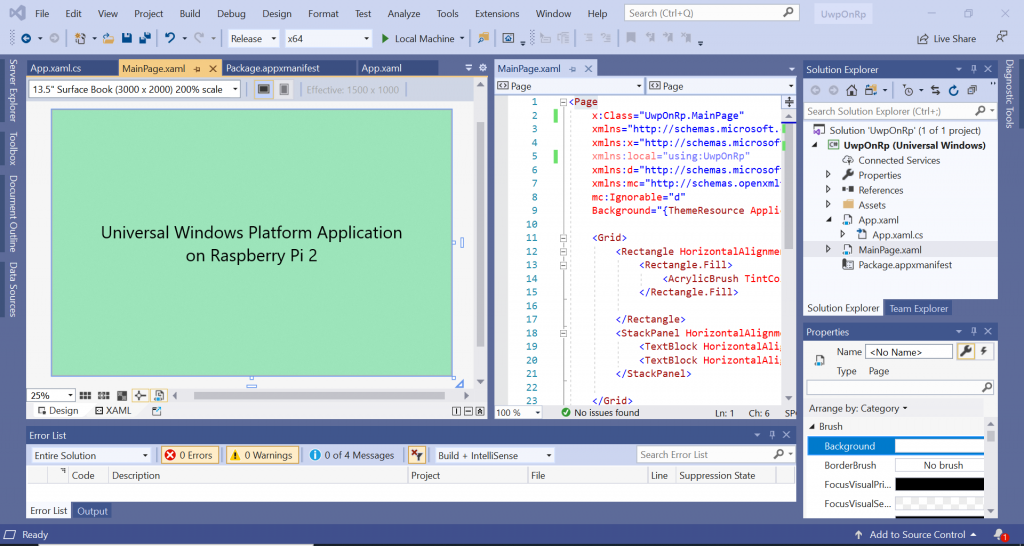
Install Windows 10 IOT Core on Raspberry Pi 2B and connect the device to a 50″ screen TV.
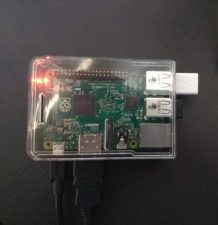
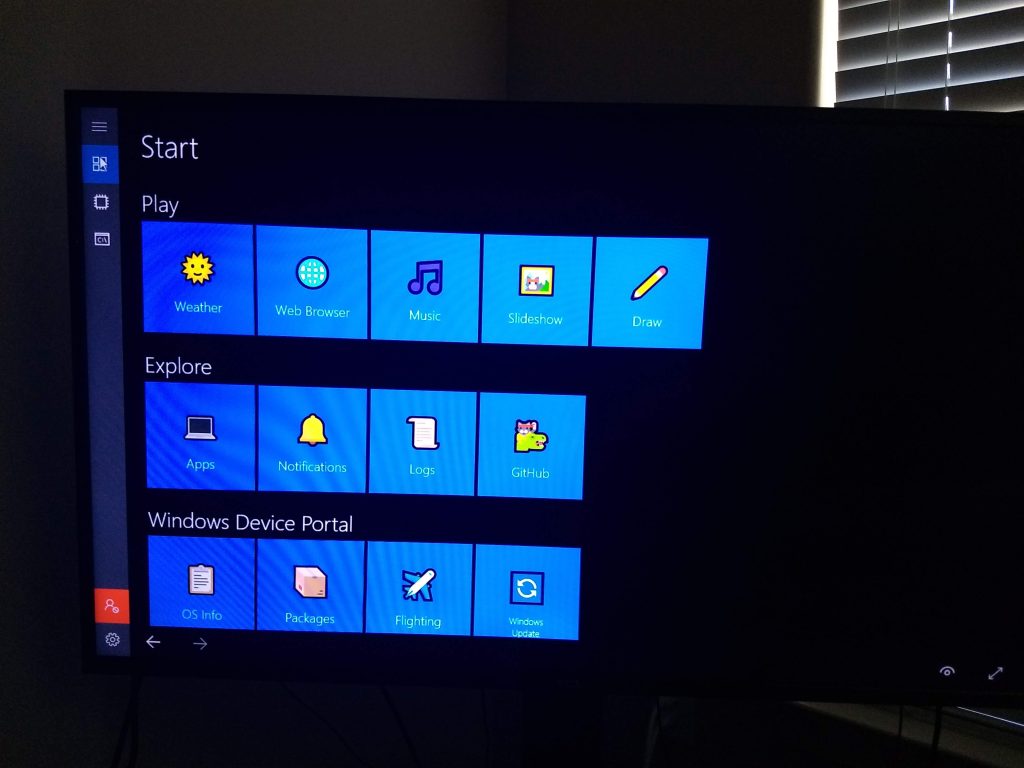
Select ARM in the target platform drop down and Click on Remote Machine to Run/Debug Application on RPi device.
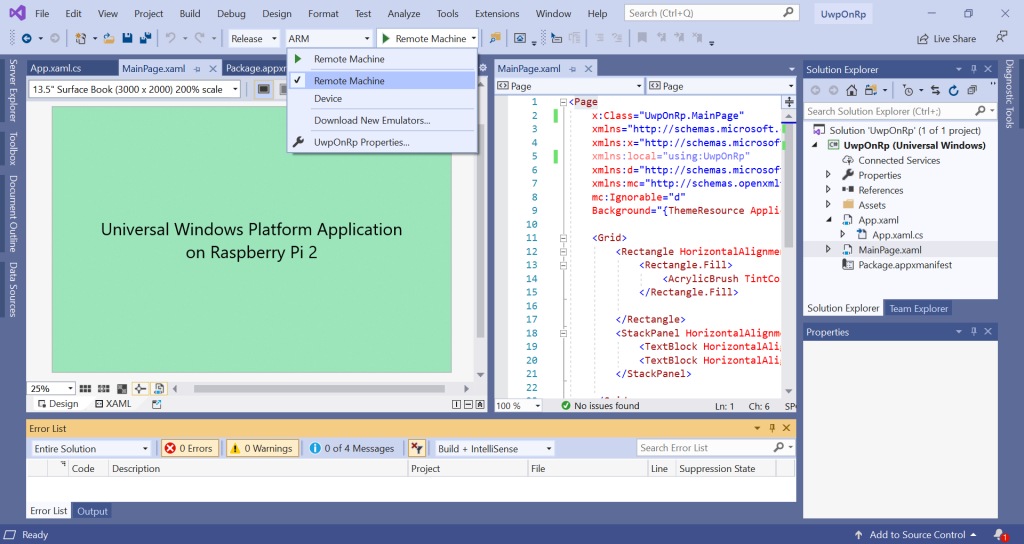
Select RPi device for deployment.
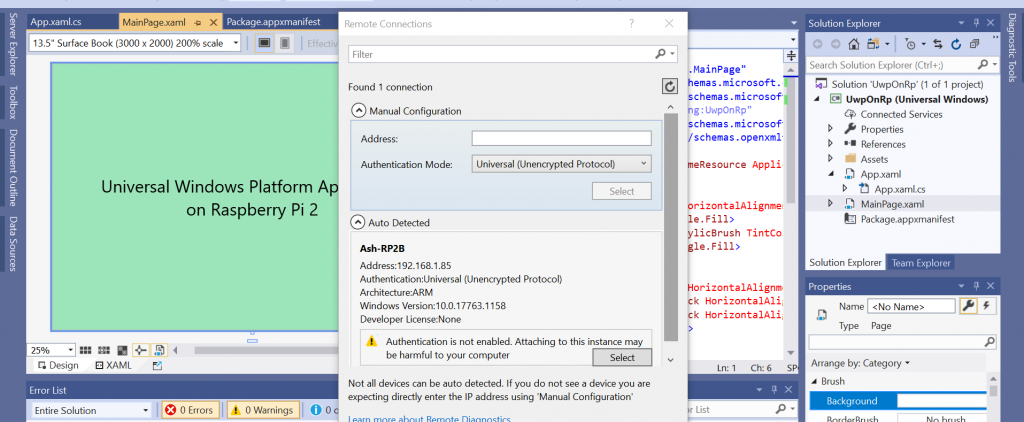
Deployment of UWP on RPi is complete.
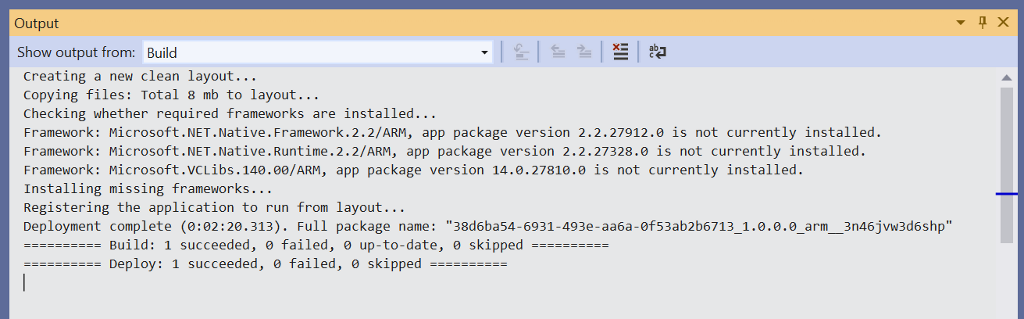
UWP application running on RPi.
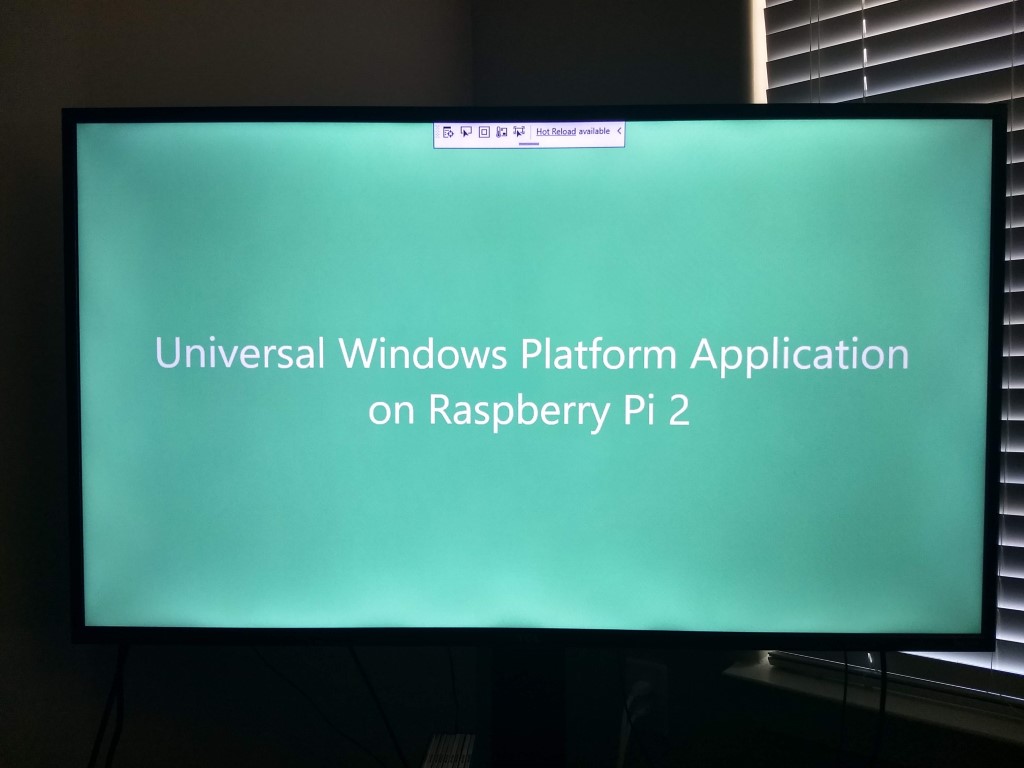
I updated the UWP application to include Syncfusion Chart control. Did you know that you can get a Community License for the entire product line (including Blazor, Xamarin and UWP controls) from Syncfusion?
UWP application is now running on RPi, attached to the Visual Studio debugger running on the development machine (Windows Surface).
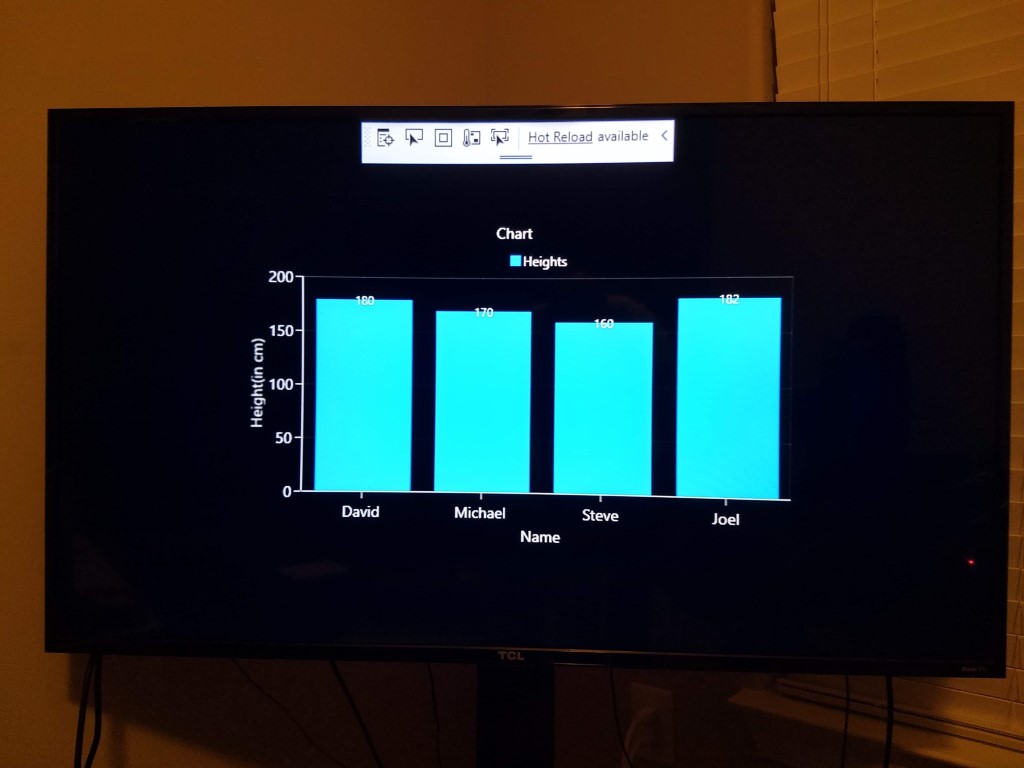
No device specific extension SDKs were referenced, just the core UWP APIs were used.
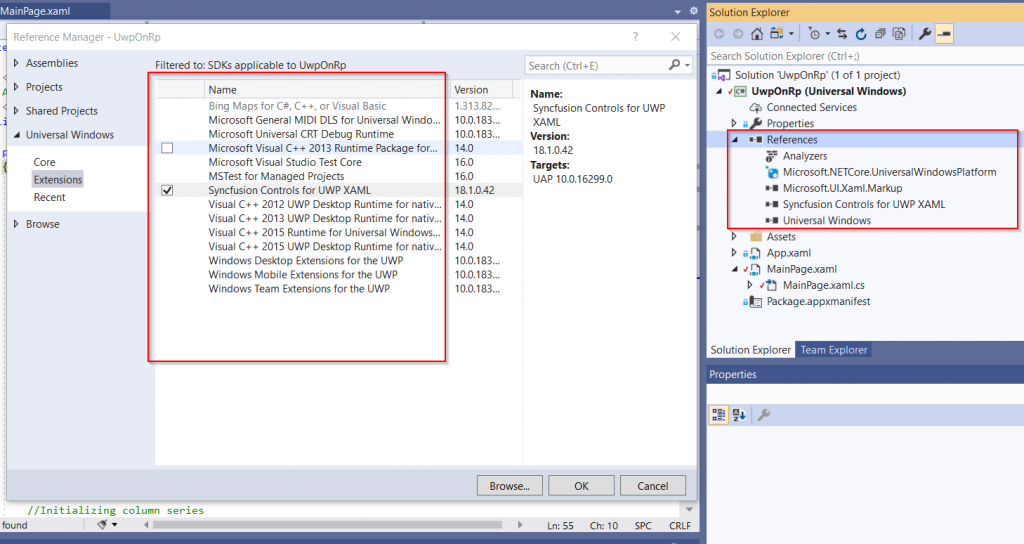
Code is on github.