You can create native Android and iOS applications using C#, with Xamarin. I explored the capabilities of Xamarin tools and the development experience with Xamarin to create an Android version of CensusMapper. CensusMapper displays U.S. Census data on a Map. I created the original application for Windows 8 (WinRT) using C#. I have since converted it to an MVVM (C#) application, HTML/Javascript (WinJS) version and more recently to a Universal Windows App running on Windows Phone 8.1 and Windows 8.1. In that tradition, now it is faithfully ported to run on Android devices, using C# and Xamarin. CensusMapper is available on Google Play Store.
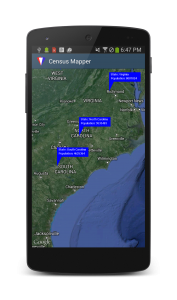
CensusMapper.Android shows population of each U.S. State. User can tap on a location on the map to show the population (as per 2010 census) of the zip code containing the selected location. User can select a location to view the population compared to the containing geography, as a pie-chart.
This experimental project helped me expand my cross-platform mobile application development skillset in the following areas:
- Using Xamarin/C# to create an Android app using Xamarin Studio on a PC and a Mac.
- Structuring C#/.NET source code in a Portable Class Library to allow reuse on Windows, Windows Phone, Android and iOS platforms.
- Converting the functionality of an existing Windows Universal App (Windows Phone/ WinRT) to an Android app.
- Using GoogleMaps API (via Google Play Services) to display data retrieved from U.S. Census API.
- Using Bing Maps API for reverse geocoding in an Android app.
- Creating custom markers using low-level API in Android.Graphics namespace to display information on the map.
- Charting with oxyplot cross-platform charting library.
- Working with Android SDK, AVD Manager as well as developer documentation and resources on Android and Xamarin websites in the development lifecycle of the app.
- Publishing an app on Google Play Store.
I started with Xamarin.Forms.Maps. It was quick and straight forward to create a Xamarin.Forms app. The tricky part was creating the custom markers (the shape of speech bubble) on the map, instead of using the default push-pin markers . I tried creating a CustomRender to create custom markers and built interactivity with the map elements, but it turned out to be too much hassle. All of that customization would be specific to Android platform only and not reusable on the iOS anyway. So, I decided against using Xamarin.Forms and work directly with Google Maps library. Xamarin.Forms also limits me to Windows Phone 8 compatibility in my PCL. I can’t use Windows Phone 8.1 in any PCL profile compatible with Xamarin.Forms. It also requires a minimum of Android 4.0. The PCL profile # 111 for sharing code between Windows 8, Windows Phone 8.1 as well as Android and iOS, is not compatible with Xamarin.Forms at the time of writing this.
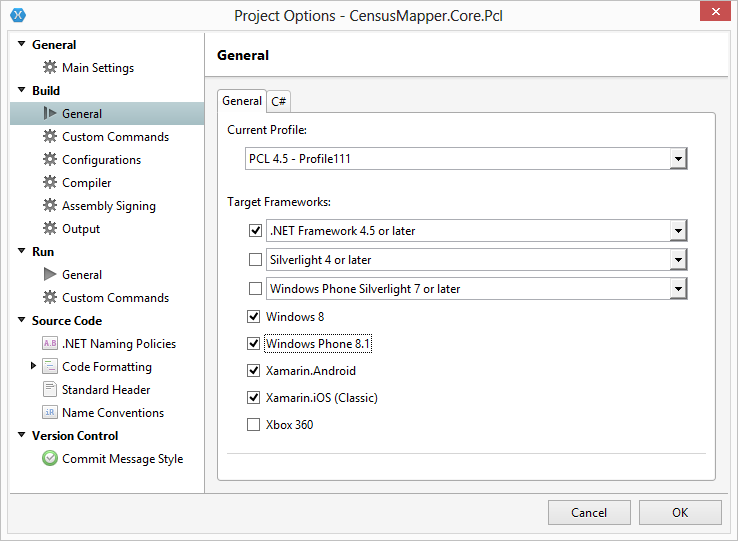
Don’t use the Android emulator for testing. Get a real device. I stopped using the emulator while working on CensusMapper because GooglePlayServices (GPS) dependency was making me jump through way too many hoops. Debugging map functionality on the real device was a much more productive experience. Then I discovered Xamarin’s Android Player. It is awesome. By all means, use it! It is a pleasure to work with, especially on a Mac. Don’t be discouraged by the preview/beta status. It blows the Android SDK virtual devices out of the water, in terms of performance and usability.
I tested on Google Nexus 4 (Android 4.2), Samsung Galaxy SIII (Android 4.3) , BLU Dash JR (Android 4.4) and an older Coby Kyros tablet (Android 2.2). Google Play Services update, required for the app to work, could not be installed on BLU device because of the limited storage space. You must root the device in order to install the GPS updates on an SD card. It is advisable to have a root-friendly device. Google Nexus devices are excellent development devices, allowing you root access and the ability to load custom ROMs. However, it is very important for you to get a few different kinds of devices of various form factors and Android versions/API levels for testing your app’s functional and non-functional aspects.
Android device logs and the IDE build logs are the most important tools you have to resolve problems encountered in the Android app development with Xamarin. These should be the first places to look when troubleshooting issue.
Versions used in this project:
Xamarin 3.8.
Xamarin Studio 5.7
Xamarin Android 4.20.0
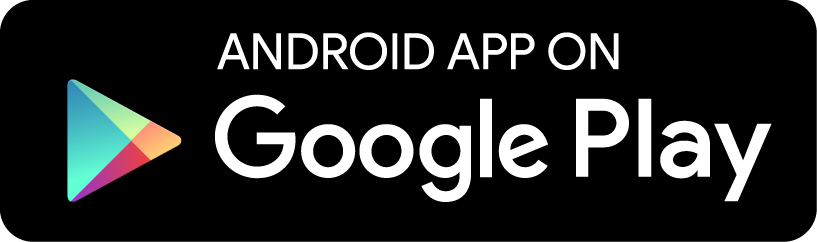